Visualizing Location-based online social networks
Goal
- Explore location-based datasets from SNAP (using loc-gowalla in this post)
- Learn how to draw maps using R (from StackOverflow and Google haha)
Downloading the dataset
Download the dataset, including the social network and check-in logs. Save the introduction of this dataset.
1 |
|
Visualization
I put all codes at the end of this post.
At first glance
I first visualize check-in logs in a map (see ref. [1]). Because there are too many check-in logs, I only randomly sample some to them to visualize. The output is as follows. We can see that most logs are check-ins to the US or countries in Europe.
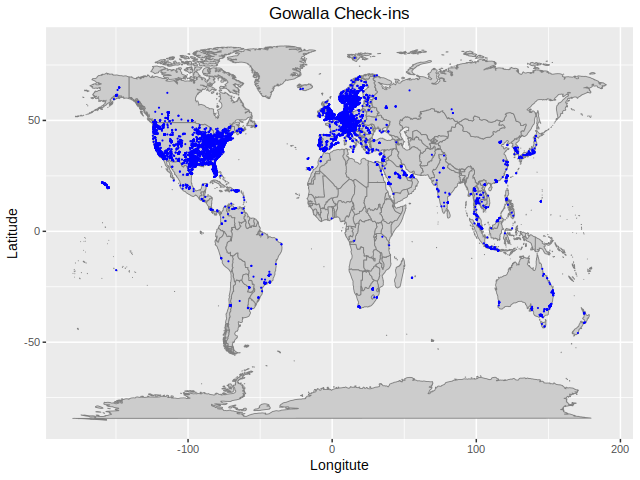
Mapping GPS to detailed locations
Mapping country codes to check-ins is done by the method in [2]. For simplicity (because I am lazy), I only keep the country name of each check-in. Countries with most check-ins are listed as follows.
1 | country Freq |
Visualizing check-ins of each country/area
Check-ins have been labeled by their corresponding country/area in the previous step. I visualize the total number of check-ins of each country. The results are as follows.
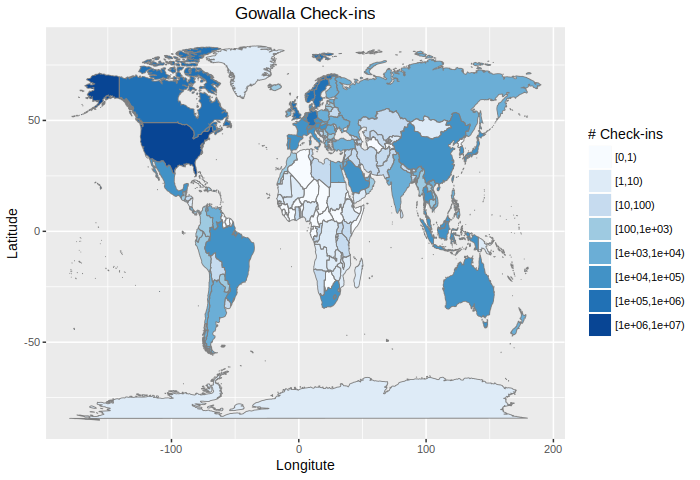
Visualizing check-ins of the US
Because more than half of the check-ins are map to locations in US. We visualize the number of check-ins to each state of the US. Only lower 48 states and Washington, D.C. are shown in the figure. (The “map_data(‘state’)” only contains lower 48 states + D.C.) The results are as follows.
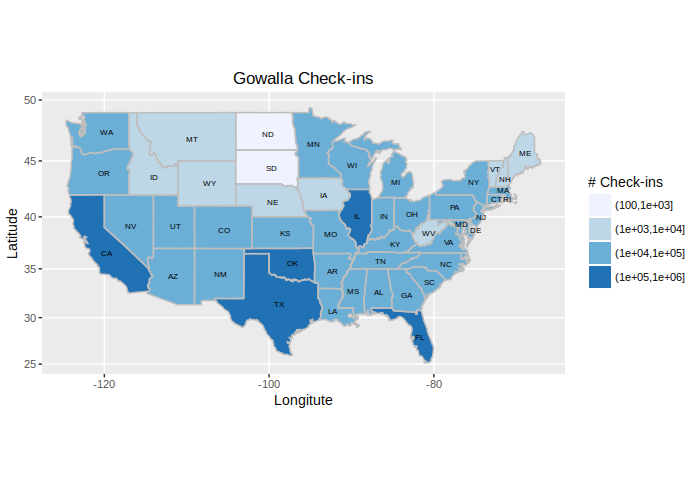
In fact, top-5 states with most check-ins are as follows.
1 | region Freq |
Codes
Codes are as follows. I hope they are self explanatory.
1 | library(data.table) |
References
- R Beginners – Plotting Locations on to a World Map https://www.r-bloggers.com/r-beginners-plotting-locations-on-to-a-world-map/
- Assign Country Code to Tweets Based on GPS Coordinates https://zacharyst.com/2016/02/12/when-twitter-doesnt-give-a-country-code/
- Administrative regions map of a country with ggmap and ggplot2 (Visualizing unemployment rate) http://stackoverflow.com/questions/17723822/administrative-regions-map-of-a-country-with-ggmap-and-ggplot2